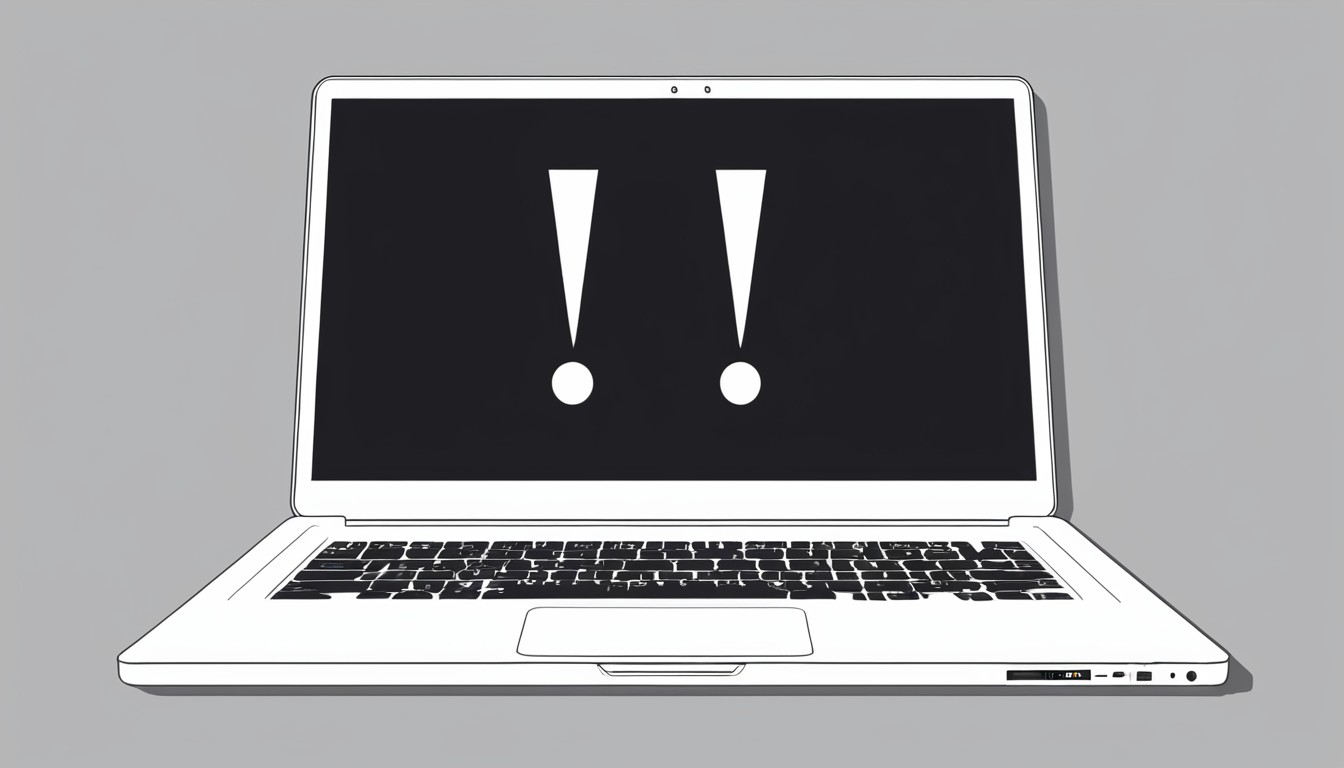
You’ve probably seen it lurking in the shadows of some JavaScript code snippets — but its cryptic nature may have left you scratching your head. In JavaScript, the double exclamation mark (!!) is a unary operator that converts the operand into its equivalent boolean value. It does this by first casting the operand to a truthy or falsy value and then negates it twice.
// Log the boolean equivalent of each value
console.log(!!0); // Output: false
console.log(!!1); // Output: true
console.log(!!''); // Output: false
console.log(!!'Hello'); // Output: true
console.log(!!null); // Output: false
console.log(!!undefined); // Output: false
console.log(!!NaN); // Output: false
console.log(!!false); // Output: false
console.log(!!true); // Output: true
console.log(!![]); // Output: true
console.log(!!{}); // Output: true
console.log(!!(() => {})); // Output: true
In this code snippet, each value is placed directly inside the console.log()
call, and the !!
operator is used to convert each value to its boolean equivalent. The result is then logged to the console.
Understanding the Basics
A singular exclamation mark (!), also known as the logical NOT operator. This operator is used to reverse the boolean value of an operand.
let isOpen = true;
console.log(!isOpen); // Output: false
In the above example, isOpen
is initially true
. When we apply the !
operator, it reverses the value, resulting in false
.
Truthy and Falsy Values
In JavaScript, values are not just true
or false
. They can also be “truthy” or “falsy”. These are values that aren’t actually true
or false
, but behave like them when evaluated in a boolean context.
Here are the falsy values in JavaScript:
false
0
(zero)''
or""
(empty string)null
undefined
NaN
document.all
(Don’t worry, this is the only JavaScript object that’s considered falsy)
Everything else in JavaScript is considered truthy.
Now, this is where the double exclamation mark comes into play. By using !!
, we can explicitly convert any value to a boolean equivalent – true
or false
.
let name = 'John';
console.log(!!name); // Output: true
In this example, the string ‘John’ is a truthy value. When we use !!
, it converts the truthy ‘John’ to a boolean true
.
Practical Uses of Double Exclamation Mark
The !!
operator is incredibly useful in scenarios where we need to check the existence or absence of a value.
Let’s look at an example where we want to check if a user has input their name:
function greetUser(name) {
if (!!name) {
console.log(`Hello, ${name}!`);
} else {
console.log('Hello, Guest!');
}
}
greetUser('John'); // Output: Hello, John!
greetUser(''); // Output: Hello, Guest!
In this greetUser
function, we use !!name
to check if the name
parameter exists. If name
holds a truthy value (i.e., a non-empty string), the function greets the user by name. If name
is falsy (i.e., an empty string), the function defaults to greeting a ‘Guest’.
The !!
operator can also be handy when working with optional properties in objects:
let user = {
name: 'John',
age: 30,
// isAdmin: true
}
if (!!user.isAdmin) {
console.log('User is an admin');
} else {
console.log('User is not an admin');
}
// Output: User is not an admin
In this case, isAdmin
property is optional. By using !!user.isAdmin
, we can easily check whether the user is an admin and handle the situation accordingly.
Be Mindful of the Pitfalls
While the !!
operator is a powerful tool, it’s important to remember that it coerces all truthy values to true
and falsy values to false
. This can sometimes lead to unexpected results.
For example, consider the following:
let emptyArray = [];
let emptyObject = {};
console.log(!!emptyArray); // Output: true
console.log(!!emptyObject); // Output: true
Even though both the array and the object are empty (which might conceptually be considered as ‘nothing’ or ‘absent’), they’re still both objects in JavaScript and therefore a truthy value. Hence, !!
converts it to true
.
Also, remember that null
, undefined
, and NaN
are all falsy values in JavaScript. So if you’re using !!
on a value that could potentially be null
or undefined
, make sure your code can handle these cases correctly.
console.log(!!null); // Output: false
console.log(!!undefined); // Output: false
console.log(!!NaN); // Output: false
Conclusion
The double exclamation mark in JavaScript is one of those small but useful tools in your kit. It provides a clear and concise way to convert any value into a boolean, making your code more expressive and predictable. However, it’s not a silver bullet. As with any programming construct, understanding its limits and its interplay with other parts of the language is key to using it effectively.
The next time you’re working on a JavaScript project, consider the potential for the double exclamation mark to simplify your conditionals and improve the clarity of your code. Just remember, with great power comes great responsibility — and in the realm of JavaScript, code that’s readable and maintainable is just as important as code that works.