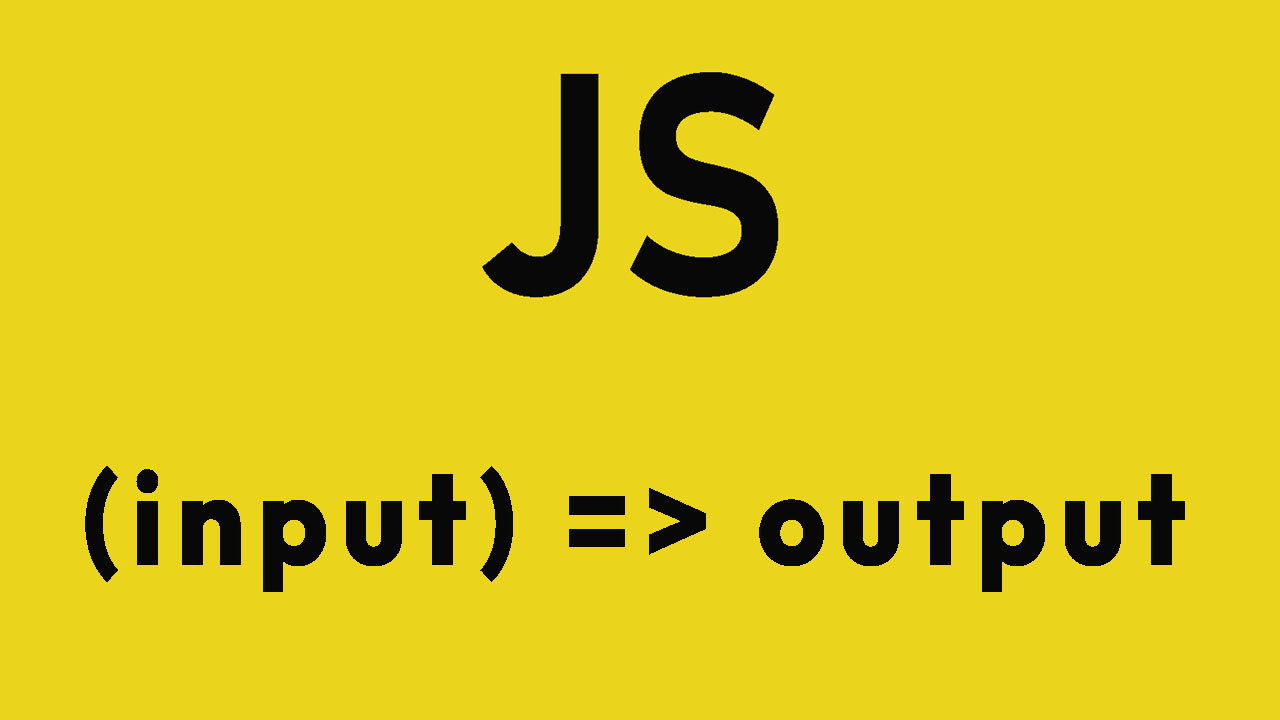
Javascript functions are very flexible compared to other programming languages. They can be declared in many ways, they can look very different in the code, and they can be referenced and passed throughout the code. But in this post, I would like to focus on the 3 main types of functions in Javascript: Named functions, Anonymous Functions, and Arrow Functions.
Named Functions
Named functions are a standard function type where the function is declared with the function
keyword followed by a unique name. Named functions can be called both before and after they have been defined due to JavaScript’s function hoisting. Let’s look at the syntax:
function greet() {
console.log('Hello, world!');
}
greet(); // Outputs: 'Hello, world!'
In this example, greet
is the name of the function. We then used that name to call the function on line 5. Named functions are the most well-known type of Javascript functions. They are excellent for reusability and readability, especially when your codebase becomes larger.
Anonymous Functions
Unlike named functions, anonymous functions don’t have a specific name. They are usually stored in a variable or used as a callback function. Here’s a common syntax of an anonymous function:
let greet = function() {
console.log('Hello, world!');
}
greet(); // Outputs: 'Hello, world!'
Instead of naming the function, we created an anonymous function and assigned it to a variable called greet
. We then used the variable name to invoke the function.
You might think that this is no different than named functions. And in the example above, you would be right. But anonymous functions can also be passed as callbacks like so:
let greet = function(callback) {
console.log('Hello!');
callback();
}
greet(function() {
console.log('How are you?');
});
The code above will output the following:
Hello!
How are you?
In this example the callback function doesn’t have a name, we didn’t even assign it to a variable, but we injected it directly as an argument of the greet
function. The greet
function then called the callback function.
Anonymous functions are great for one-time tasks or as arguments to other functions where the function’s logic is not reused elsewhere in the code. Although we are seeing an increase in the usage of anonymous functions assigned to variables as an alternative to named functions. You can pick the one that you like, it’s a matter of personal preference.
Arrow Functions
Arrow functions, introduced in ES6, offer a more concise syntax for writing functions. They are also anonymous, but they have some differences in functionality, such as not having their own arguments
object, and not creating their own this
binding. Here’s an example of an arrow function:
let greet = () => {
console.log('Hello, world!');
}
greet(); // Outputs: 'Hello, world!'
Arrow functions are excellent when you want to write shorter function syntax and when you want this
to be bound to the surrounding code’s context. For more information about the differences in functionality between arrow functions and regular functions, I recommend checking out this amazing article on Free Code Camp.
The Most Used Function Type
In the last few years, I have seen a clear trend of Javascript developers starting to prefer the usage of anonymous arrow functions, and for good reason. I think that arrow functions provide a more concise syntax and address several quirks associated with traditional function expressions, particularly around the behavior of this
. They’re incredibly handy for writing shorter function expressions and they are widely used in modern libraries and frameworks such as React and Vue.js. While they’re not suited for all uses, for example, they cannot be used as object constructors, their benefits in creating cleaner, more readable code have led to their increasing adoption in the JavaScript community.
Final Notes
In JavaScript, understanding the differences between named functions, anonymous functions, and arrow functions is key to writing efficient and readable code. Named functions provide reusability and can be hoisted, allowing them to be used before they are defined. Anonymous functions are great for one-off tasks and as arguments to other functions. Arrow functions provide a shorter syntax and do not have their own this
binding, making them ideal for certain use cases.
The right one to use largely depends on the specific scenario and the structure of your code. The more you code and experiment with these functions, the more intuitive choosing the right one will become. Happy coding!